RESTful CRUD Api using Entity Framework Core's In-Memory (O/RM) Database - C# ASP.Net Core Web Api
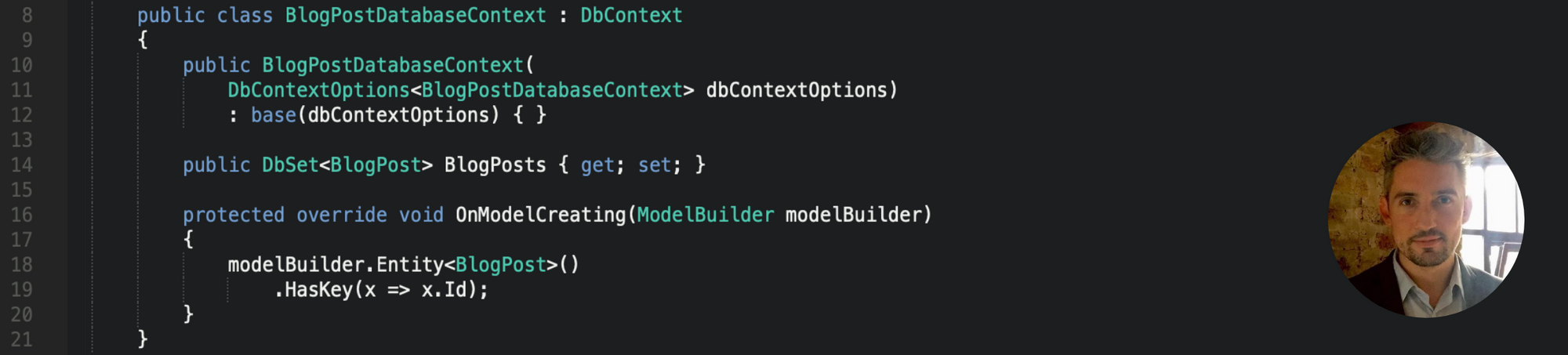
Use Case
I recently needed to implement a CRUD layer in a RESTful Api, however the decision regarding which database context to use to store our data hadn’t quite happened. Given this, I decided to build an abstract implementation which would allow us to “plug-and-play” our chosen database framework into the Api at a later date.
Rather than going down the route of spinning up a cloud database to test out the abstraction, I decided to use an in-memory database context…
Enter “Microsoft’s Entity Framework Core”…
“Entity Framework (EF) Core is a lightweight, extensible, open source and cross-platform version of the popular Entity Framework data access technology. EF Core serves as an object-relational mapper (O/RM), enabling .NET developers to work with a database using .NET objects, and eliminating the need for most of the data-access code they usually need to write.”
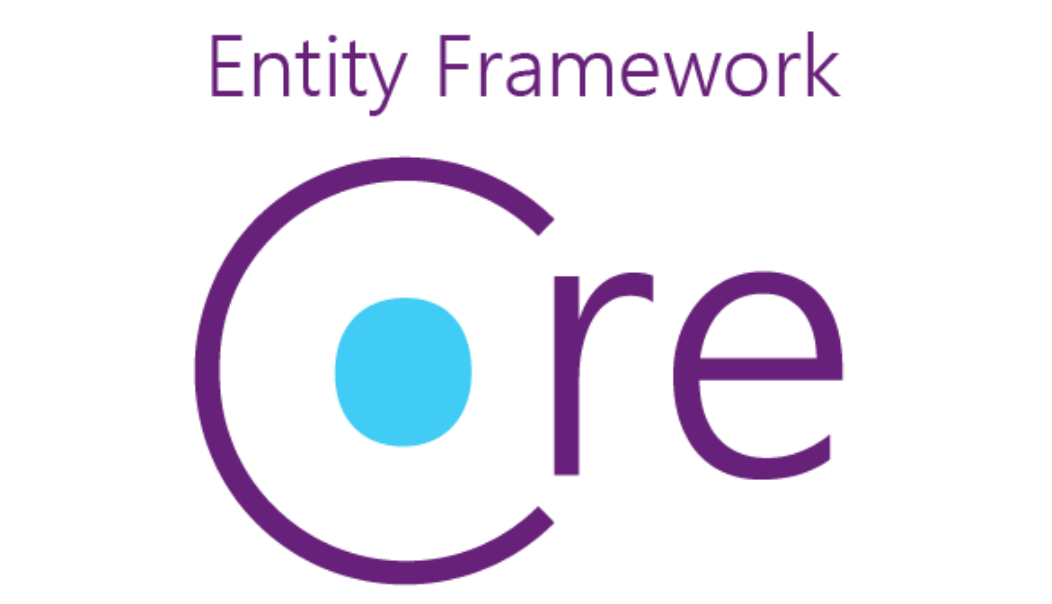
Entity Framework Core’s In-Memory (O/RM) Database - C# ASP.Net Core Web Api
If you’ve ever had the luxury of using the Entity Framework you’re already well aware of how capable it is and how awesome O/RM is, not to mention how clean the implementation is to work with. Fortunately they now support .Net Core, so let’s give it a whirl and build a RESTful CRUD Api using the Entity Framework In-Memory Database!
Let’s Code…
Today’s example is based on storing a series of blog posts via a RESTful CRUD Api. First, create a fresh ASP.Net MVC Core project and then pull in a copy of the relevant package from Nuget.

Entity Framework Core’s In-Memory (O/RM) Database - C# ASP.Net Core Web Api
Our abstraction will work as follows: Controller > Service > Repository > Database Context
Let’s start by defining a data model to use -
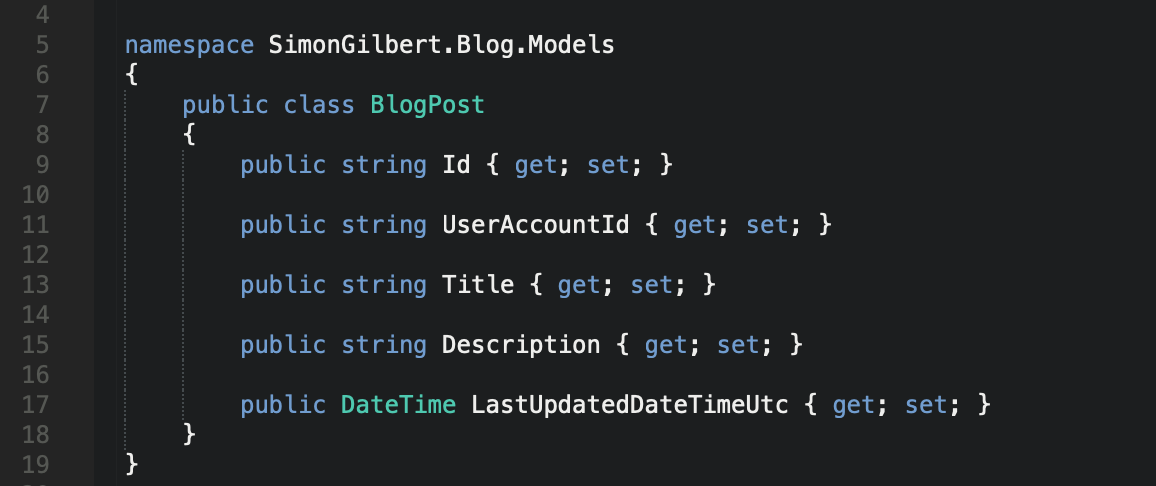
Entity Framework Core’s In-Memory (O/RM) Database - C# ASP.Net Core Web Api
Next we need to code our database context layer by defining our database table for our blog post entities, and setting our primary key.
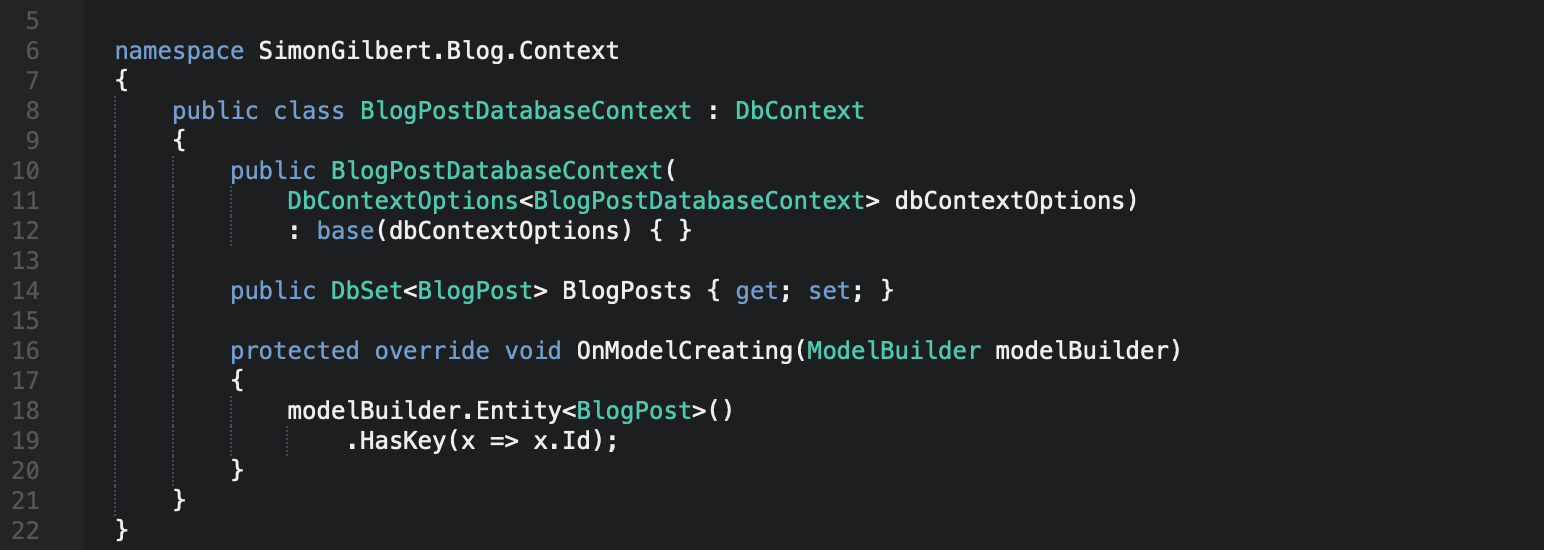
Entity Framework Core’s In-Memory (O/RM) Database - C# ASP.Net Core Web Api
Now to inject our database context into our repository via ServiceScope.
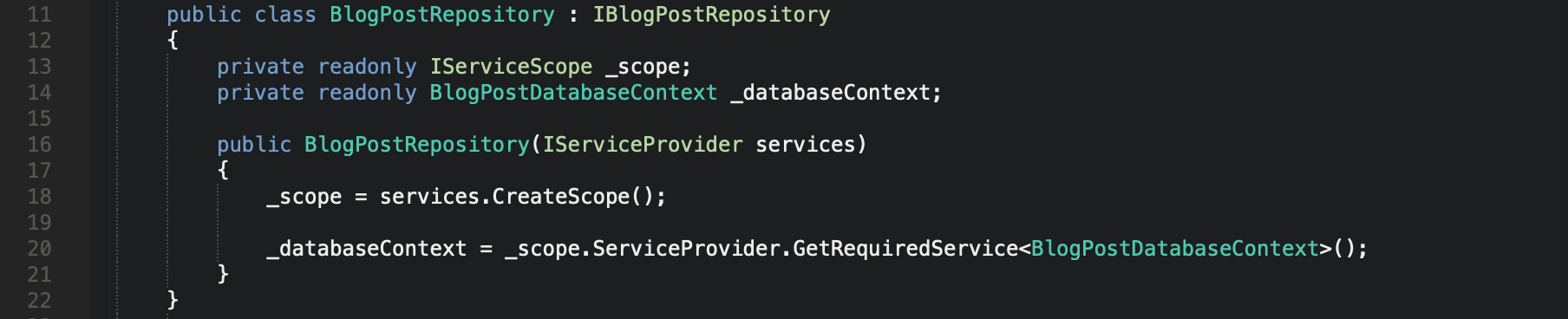
Entity Framework Core’s In-Memory (O/RM) Database - C# ASP.Net Core Web Api
We can now add a basic “create” method to our repository.
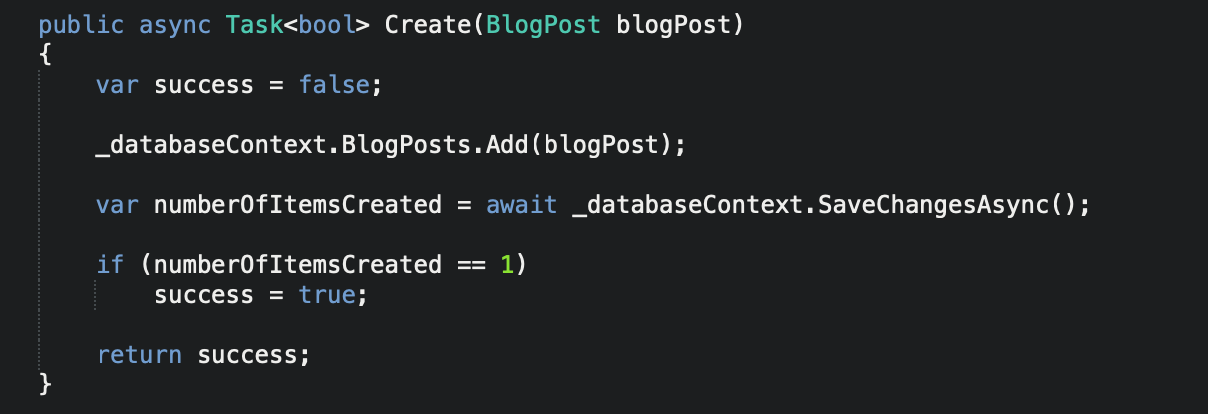
Entity Framework Core’s In-Memory (O/RM) Database - C# ASP.Net Core Web Api
Next we inject our repository into our service layer, along with a “create” method which binds to the equivalent repository method.
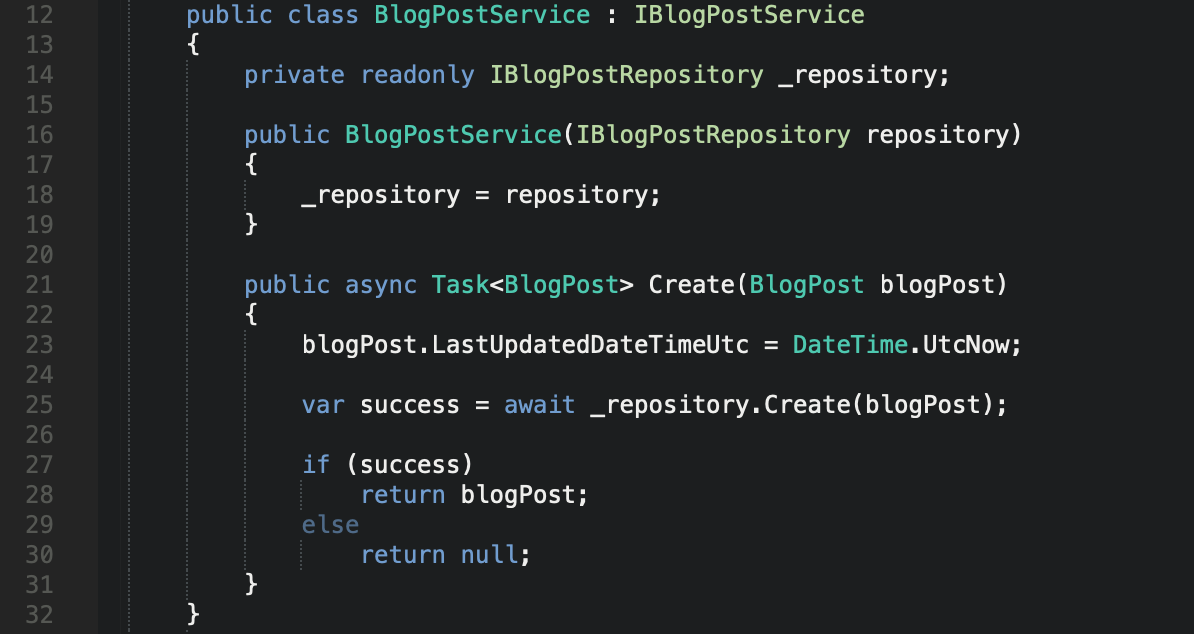
Entity Framework Core’s In-Memory (O/RM) Database - C# ASP.Net Core Web Api
Now we can inject our service into our controller.
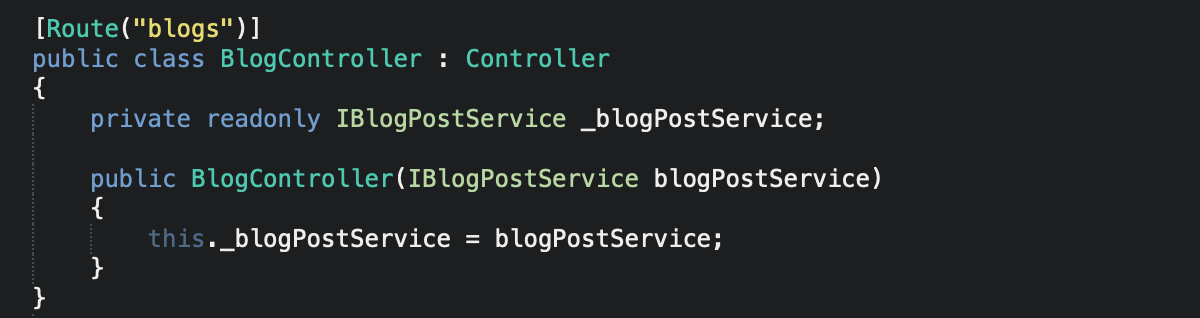
Entity Framework Core’s In-Memory (O/RM) Database - C# ASP.Net Core Web Api
…Followed by our controller action for our “create” process flow, along with some simple validation via FluentValidation.
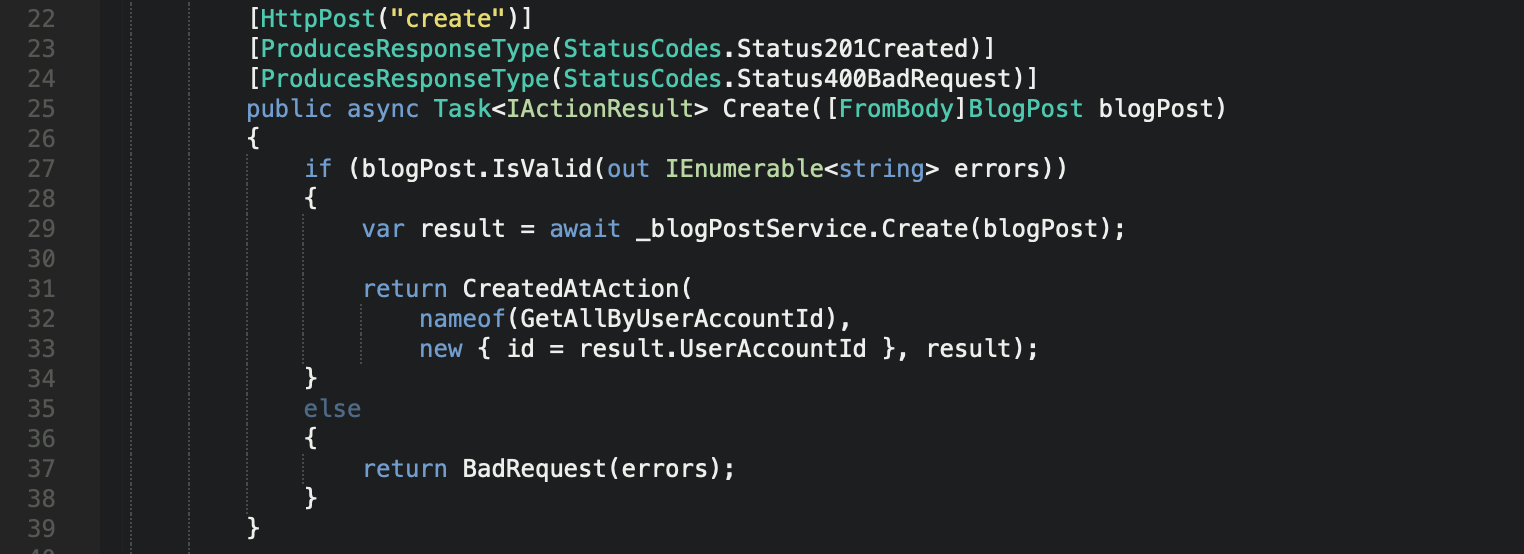
Entity Framework Core’s In-Memory (O/RM) Database - C# ASP.Net Core Web Api
Finally we can configure our dependency injection within our Startup.cs file.
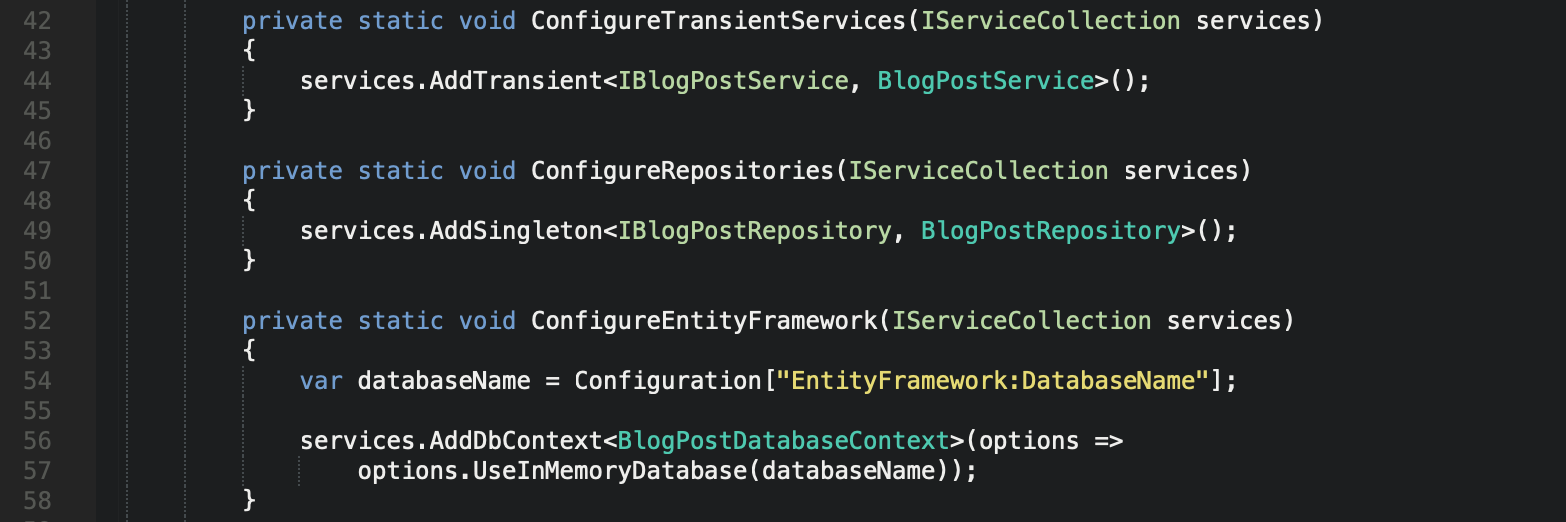
Testing Our Implementation
Let’s fire up Postman and submit data to our Api endpoints…
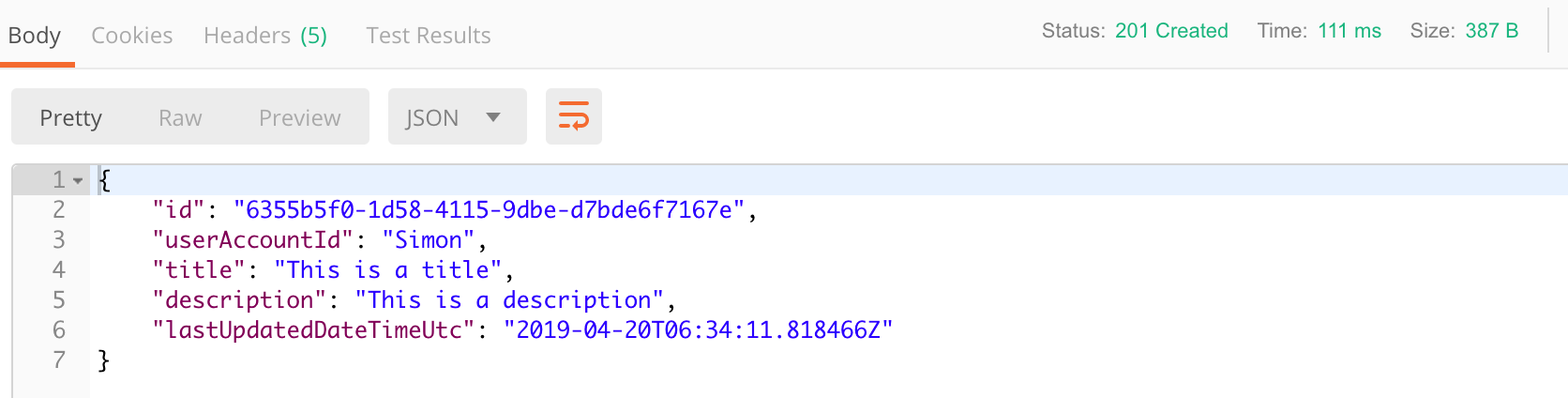
…Result!
Enjoy!